Overview
WatchOver is a gamified desktop task manager.
Summary of contributions
-
Major enhancement: added game mode functionality.
-
What it does: allows the user to switch between game modes, which are schemes for awarding XP to the user in the context of the gamified task manager.
-
Justification: This feature allows for a more dynamic experience tailored to each user. Different users are motivated by different kinds of reward schemes. Some users may prefer to have incentives for completing tasks earlier or later.
-
Highlights: This enhancement required modifying the base model. As a result, many changes had to be made throughout the internal structure of the code. There were also significant design choices in how to implement the data structure of the game mode, in order to maximise ease of use as well as future extensibility.
-
-
Code contributed: [RepoSense]
-
Other contributions:
-
Project management:
-
Was one of two repository maintainers, tasked to help manage pull requests, issues, etc.
-
-
Enhancements to existing features:
-
Refactored
-
-
Documentation:
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Change game mode: mode
Used with no arguments, mode
displays the current game mode. If used with arguments, it sets the game mode
and difficulty level.
Difficulty levels
When tasks are completed, they award xp based on criteria unique to each game mode. However, the variance of xp changes with the difficulty level. Lower difficulty levels award xp in a smaller range, whereas harder difficulties have a higher variance and are therefore riskier.
Easy
Easy difficulty awards a minimum of 40 and a maximum of 50 xp.
Medium
This is the default difficulty level, awarding a minimum of 30 and a maximum of 60 xp.
Hard
Hard difficulty awards a minimum of 20 and a maximum of 70 xp.
Extreme
Extreme difficulty awards a minimum of 10 and a maximum of 80 xp.
Game modes
Flat
Flat mode is the most basic mode and is enabled by default, awarding the higher amount of xp for a task completed on time, and the lower amount of xp for overdue task.
Decreasing
The Decreasing mode is essentially an interpolation of the Flat mode. Tasks start at the higher amount of xp, and start decreasing linearly at a date close to the due date, reaching the lower value at the due time and not decreasing further. The xp awarded starts decreasing exactly 1, 3, 7 and 10 days before the due date, for easy, medium, hard and extreme modes respectively.
Increasing
The Increasing mode is the opposite of the Decreasing mode: the xp awarded begins at the lower amount, and increases as the deadline nears, plateauing at the higher value when the deadline is reached. It is recommended for motivated users who want the xp awards to reflect the urgency of the task; the reasoning being that if the current amount of xp has not yet been able to make the task 'worth it' to complete, then the amount should increase. The time interval is the same as the Decreasing mode.
Priority
The Priority mode lets the user influence the xp awarded for individual tasks. Unlike the other modes, this mode does not follow the outlined minimums and maximums, instead applying a multiplier to the priority value. The multiplier is equal to one tenth of the Flat mode xp for the same difficulty, i.e. under Medium difficulty, an overdue task will obtain priority value * 3 xp, and a task completed on time will receive priority value * 6 xp.
Format: mode [Optional: GAME_MODE] [Optional: GAME_DIFFICULTY]
, where:
GAME_MODE
can be flat
(default), decreasing
, increasing
, priority
;
GAME_DIFFICULTY
can be easy
, medium
(default), hard
, extreme
.
GAME_MODE
must be specified in order to also set GAME_DIFFICULTY
.
+Example: mode
+Example: mode flat
+Example: mode decreasing hard
-Example: mode extreme
-Example: mode edna
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Game Manager
The Game Manager is responsible for the gamification aspects of WatchOver.
Structural Overview

The Task Manager owns a GameManager
object, which handles the core logic of gamification. All xp calculations are
handled exclusively by the current GameManager
.
The GameManager
, in turn, owns a GameMode
object. GameMode
is implemented as an abstract class, and specific
implementations of game modes must extend the abstract GameMode
. The GameMode
handles the actual appraisal of tasks
for xp, delegated from the GameManager
. Individual variations of GameMode
will contain their own logic to determine
xp earned, accessible through appraiseXpChange(Task taskFrom, Task taskTo)
.
Mechanism
When a task is completed, ModelManager::updateTaskStatus(Task target, Task updatedTask)
is used to change the
target task into the updated task. TaskManager::appraiseXpChange(target, updatedTask)
is then called (from
the current versionedTaskManager
instance).
At this point, the TaskManager
calls GameManager::appraiseXpChange(Task taskFrom, Task taskTo)
, passing
control to the gamification component.
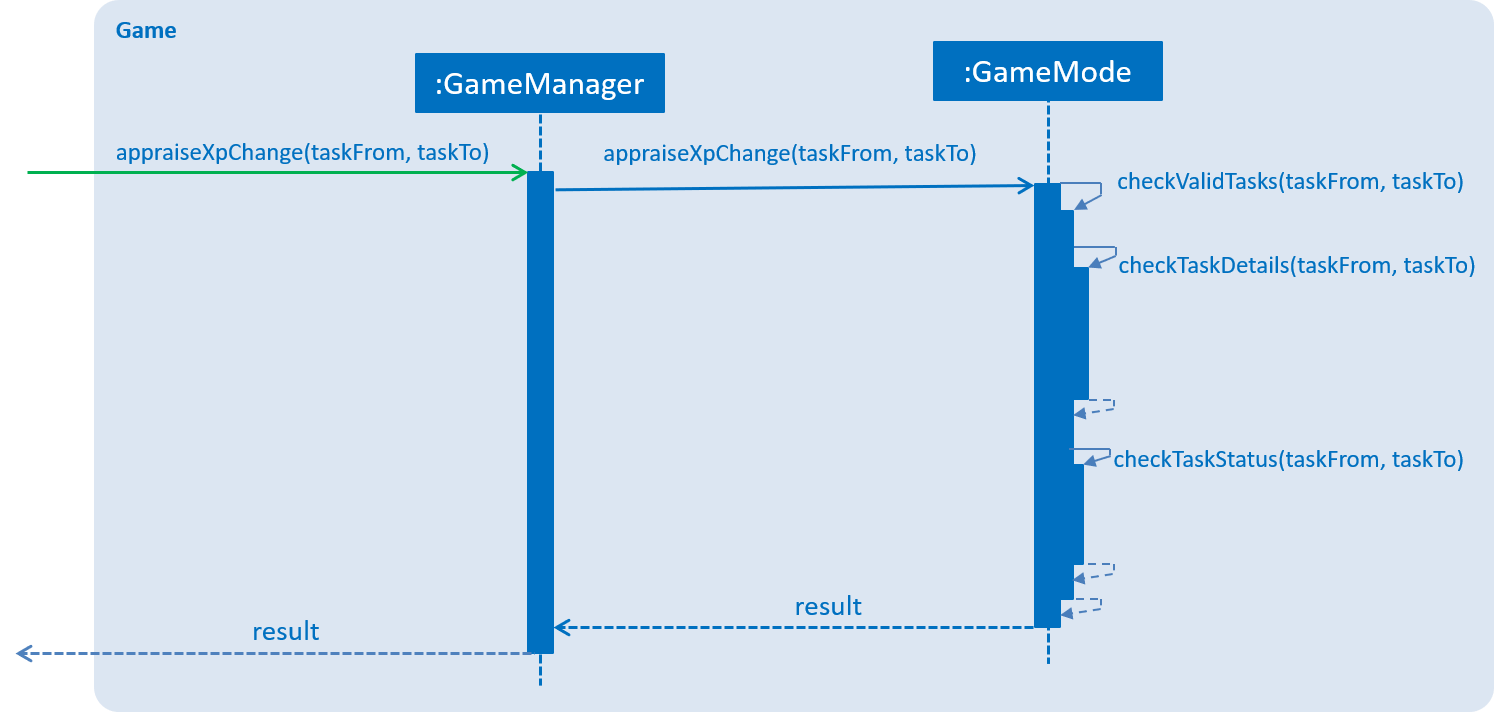
The GameManager
delegates the xp calculation to its owned GameMode
through
GameMode::appraiseXpChange(Task taskFrom, Task taskTo)
. The GameMode
first checks if the tasks are valid. In
particular, the tasks must be the same tasks (that is, they have the same fields), and the status change must be
a valid change (e.g. from IN_PROGRESS
to COMPLETED
, and not something like COMPLETED
to OVERDUE
).
Following that, the GameMode
performs the specific calculations unique to each GameMode
, to determine the xp
to be awarded.
Storage
The current GameManager
is persisted in WatchOver’s storage.
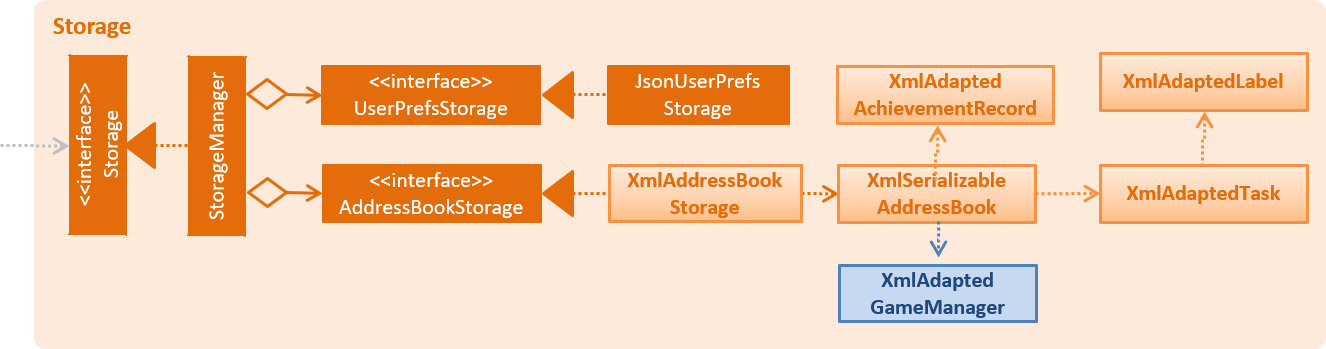
The XmlSerializableTaskManager
contains a XmlAdaptedGameManager
, to capture the state of the GameManager
,
allow persisting state between sessions, and take advantage of the version control to allow undoing and redoing
of changing the game mode.
Four fields are written to file:
-
the game mode,
-
the period,
-
the lower bound of xp awarded, and
-
the higher bound of xp awarded.
The game mode is serialised as the SimpleName of the GameMode
subclass in the stored GameManager.
The period is the time before the due date at which the xp awarded by DecreasingMode
and IncreasingMode
will start
to fall or rise, respectively. Other modes ignore this field.
The lower and higher bound of xp awarded are the scalings to which the game modes peg their xp awards, used by all existing game modes.
Using these fields, the GameManager
state can be fully reconstructed.
If an invalid game mode is found written to storage, then the invalid data is ignored and a new default GameManager
,
using the default FlatMode
set at medium difficulty, will be created, overwriting the invalid data.
Game Manager Design Considerations
Aspect: Location of xp Calculation Logic
-
Alternative 1 (current choice): Using a separate GameManager class to calculate awarded XP.
-
Pros: It is modular and isolated from the rest of the logic, resulting in higher maintainability.
-
Cons: Less flexibility, and will apply flatly across all tasks, with no window for individual variation.
-
-
Alternative 2: Embedding XP calculation logic within task completion logic.
-
Pros: XP awards is primarily used when completing tasks. Placing code here would have relevant code closer together.
-
Cons: It is not modular, resulting in higher coupling and lower maintainability.
-
-
Alternative 3: Embedding XP calculation logic inside each individual task.
-
Pros: This would allow users to set different modes for each individual task, rather than having a one-size-fits-all policy apply to all tasks.
-
Cons: Difficult for user to edit the modes of all tasks at one shot. Additionally, the different modes attached to individual tasks may cause user confusion, especially if the user decides to change mode later but forgets to update previously set tasks. It is best to centralise all calculations to a single type of mode.
-
Aspect: Placement of GameMode
Logic
-
Alternative 1 (current choice): Wrapping the
GameMode
inside aGameManager
-
Pros: Since not all gamification elements are tied to the game mode, this allows future extensibility by creating the scaffolding for future such features to be included, such as in
v2.0
. -
Cons: One more layer of abstraction, and additional code and tests need to be written.
-
-
Alternative 2:
TaskManager
contains aGameMode
directly, instead of aGameManager
-
Pros: There is less code to write, and the code becomes more readable.
-
Cons: The above is only true so long as
GameMode
is the only gamification feature in WatchOver. Once a new feature is added, significant restructuring needs to take place which will break many things.
-
Extensions
Creating a new GameMode
Creating a new GameMode
can be done by extending GameMode
and implementing the required abstract methods.
Note that the following areas must also be changed to accomodate the new command:
GameMode::setGameMode
must handle the new game mode.
GameMode::isValidGameMode
must recognise the game mode as an existing mode.
Additionally, if the new game mode requires additional storage, the XmlAdaptedGameManager
storage class must be
modified to accomodate the additional stored information.
Game Mode testing
-
Displaying current mode
-
Prerequisites: -
-
Test case:
mode
Expected: Current game mode details will be shown.
For example,
You are currently using the Priority mode! Completing a task will earn you 6 times the priority value as xp, or 3 times if the task is overdue.
-
-
Changing game mode
-
Prerequisites: -
-
Test case:
mode decreasing easy
mode
mode increasing extreme
mode
Expected: Description shown will be different during both invocations of
mode
.
-
-
Correct xp awarded in Flat mode
-
Prerequisites:
Set game mode by runningmode flat
-
Test case:
clear
add n/Task One t/01-01-2019 p/1 d/Some description
edit 1 t/01-01-2018
add n/Task Two t/31-12-2019 p/1 d/Some description
complete 1
complete 2
Expected:
Task One is overdue, and should award 30 xp under Flat mode, medium difficulty.
Task Two is on time, and should award 60 xp.
-
-
Correct xp awarded in Increasing mode
-
Prerequisites:
Set game mode by runningmode increasing
-
Test case:
clear
add n/Task One t/01-01-2019 p/1 d/Some description
edit 1 t/01-01-2018
add n/Task Two t/31-12-2019 p/1 d/Some description
complete 1
complete 2
Expected:
Task One is overdue, and should award 60 xp under Increasing mode, medium difficulty.
Task Two is on time, and should award 30 xp.
Setting Task Two to a different date within 3 days of the current date should award xp in between 30 xp and 60 xp.
-
-
Correct xp awarded in Decreasing mode
-
Prerequisites: Set game mode by running
mode decreasing
-
Test case:
clear
add n/Task One t/01-01-2019 p/1 d/Some description
edit 1 t/01-01-2018
add n/Task Two t/31-12-2019 p/1 d/Some description
complete 1
complete 2
Expected:
Task One is overdue, and should award 30 xp under Decreasing mode, medium difficulty.
Task Two is on time, and should award 60 xp.
Setting Task Two to a different date within 3 days of the current date should award xp in between 30 xp and 60 xp.
-
-
Correct xp awarded in Priority mode
-
Prerequisites: Set game mode by running
mode priority
-
Test case:
clear
add n/Task One t/31-12-2019 p/1 d/Some description
add n/Task Two t/31-12-2019 p/10 d/Some description
add n/Task Three t/01-01-2019 p/1 d/Some description
add n/Task Four t/01-01-2019 p/10 d/Some description
edit 3 t/01-01-2018
edit 4 t/01-01-2018
complete 1
complete 2
complete 3
complete 4
Expected:
Task One has priority value 1 and is not overdue, and should award 6 xp under Priority mode, medium difficulty.
Task Two has priority value 10 and is not overdue, and should award 60 xp.
Task Three has priority value 1 and is overdue, and should award 3 xp.
Task Four has priority value 10 and is overdue, and should award 30 xp.
-
-
Correct xp awarded in various game difficulties
-
Prerequisites: -
-
Test case:
clear
mode flat easy
add n/Task One t/31-12-2019 p/1 d/Some description
complete 1
mode flat extreme
add n/Task Two t/31-12-2019 p/1 d/Some description
complete 2
Expected:
Task One was completed in Easy mode, and should award 50 xp.
Task Two was completed in Extreme mode, and should award 80 xp.
-
-
Game mode persists between sessions
-
Prerequisites: -
-
Test case:
mode
exit
Restart application
mode
Expected: Mode should remain the same before and after exiting
-
-
Change of game mode can be undone
-
Prerequisites: -
-
Test case:
mode priority easy
mode
mode flat extreme
mode
undo
mode
redo
mode
Expected:
Undoing will return the mode to the previous Priority mode, and redoing will restore the subsequent Flat mode.
-